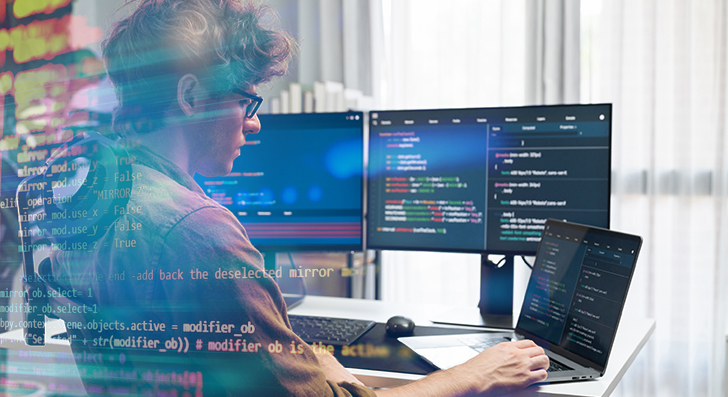
Scalability implies your application can manage development—more end users, much more data, plus more website traffic—with no breaking. As being a developer, building with scalability in your mind saves time and tension afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't a thing you bolt on later—it ought to be component of your respective strategy from the start. Numerous purposes fail when they increase quick mainly because the original style and design can’t deal with the additional load. To be a developer, you should Believe early regarding how your system will behave under pressure.
Get started by creating your architecture being flexible. Keep away from monolithic codebases where by every little thing is tightly connected. As an alternative, use modular style and design or microservices. These patterns break your application into lesser, independent elements. Just about every module or service can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day a single. Will it will need to handle 1,000,000 end users or simply just 100? Choose the correct sort—relational or NoSQL—based upon how your details will grow. Prepare for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t publish code that only will work underneath present situations. Take into consideration what would come about When your consumer foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use design patterns that help scaling, like concept queues or occasion-driven methods. These assist your app handle extra requests without the need of having overloaded.
Whenever you Develop with scalability in your mind, you are not just planning for achievement—you are decreasing future problems. A perfectly-prepared process is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Databases
Deciding on the appropriate databases is actually a important Element of making scalable programs. Not all databases are crafted exactly the same, and utilizing the Mistaken one can slow you down or even bring about failures as your app grows.
Start by knowledge your knowledge. Is it really structured, like rows in the table? If Of course, a relational databases like PostgreSQL or MySQL is an effective fit. These are typically sturdy with relationships, transactions, and regularity. They also guidance scaling strategies like study replicas, indexing, and partitioning to take care of additional targeted traffic and data.
In the event your knowledge is more versatile—like user action logs, products catalogs, or paperwork—take into consideration a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and can scale horizontally far more easily.
Also, take into account your browse and compose styles. Are you presently carrying out numerous reads with fewer writes? Use caching and read replicas. Will you be managing a large publish load? Take a look at databases that will take care of higher publish throughput, or simply event-based mostly knowledge storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Imagine in advance. You may not need to have State-of-the-art scaling features now, but choosing a database that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Stay clear of avoidable joins. Normalize or denormalize your info dependant upon your entry designs. And generally watch databases effectiveness when you improve.
To put it briefly, the ideal databases depends upon your app’s composition, velocity requires, And exactly how you be expecting it to improve. Acquire time to select correctly—it’ll preserve plenty of issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, each individual smaller hold off adds up. Poorly written code or unoptimized queries can decelerate performance and overload your system. That’s why it’s imperative that you Make successful logic from the start.
Start by crafting cleanse, basic code. Stay away from repeating logic and take away nearly anything unneeded. Don’t select the most sophisticated Answer if a straightforward one particular functions. Keep the features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—spots exactly where your code usually takes also long to operate or utilizes far too much memory.
Following, take a look at your databases queries. These frequently gradual issues down greater than the code by itself. Make certain Just about every query only asks for the information you actually need to have. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to speed up lookups. And prevent performing too many joins, Primarily across substantial tables.
If you observe a similar information staying asked for repeatedly, use caching. Retail store the outcomes briefly applying resources like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and tends to make your app far more economical.
Make sure to test with huge datasets. Code and queries that operate high-quality with a hundred documents may crash after they have to manage one million.
Briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when necessary. These measures support your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to take care of more users and much more visitors. If every little thing goes by means of a single server, it's going to swiftly become a bottleneck. That’s exactly where load balancing and caching come in. These two tools assistance keep your application speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. As opposed to 1 server performing all the work, the load balancer routes buyers to unique servers determined by availability. This implies no solitary server gets overloaded. If a single server goes down, the load balancer can send visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to put in place.
Caching is about storing information quickly so it could be reused swiftly. When users ask for the exact same details again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There's two widespread types of caching:
one. Server-aspect caching (like Redis or Memcached) stores knowledge in memory for quick entry.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves velocity, and tends to make your application more productive.
Use caching for things that don’t modify normally. And often be certain your cache is up to date when facts does alter.
Briefly, load balancing and caching are simple but strong applications. With each other, they assist your app cope with more consumers, keep fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application grow very easily. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to rent servers and providers as you require them. You don’t should invest in components or guess potential ability. When targeted traffic boosts, you could increase extra means with just some clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and stability applications. You are able to concentrate on developing your app in lieu of running check here infrastructure.
Containers are A different critical Device. A container packages your app and all the things it really should operate—code, libraries, settings—into 1 device. This causes it to be straightforward to move your application concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs several containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it routinely.
Containers also allow it to be easy to different areas of your application into companies. You are able to update or scale pieces independently, that's great for effectiveness and reliability.
Briefly, making use of cloud and container applications implies you can scale rapidly, deploy easily, and Get well quickly when troubles happen. If you prefer your app to improve with out boundaries, start employing these applications early. They preserve time, lower danger, and allow you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when things go Erroneous. Monitoring assists you see how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Section of making scalable systems.
Begin by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application far too. Control how much time it's going to take for buyers to load internet pages, how frequently faults materialize, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening inside your code.
Arrange alerts for important problems. For example, Should your response time goes above a Restrict or simply a company goes down, you'll want to get notified promptly. This can help you correct troubles quickly, usually prior to users even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again ahead of it triggers real destruction.
As your app grows, visitors and details enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and making sure it really works well, even stressed.
Remaining Ideas
Scalability isn’t only for huge providers. Even tiny applications require a robust foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without breaking under pressure. Start out small, Feel significant, and Develop good.